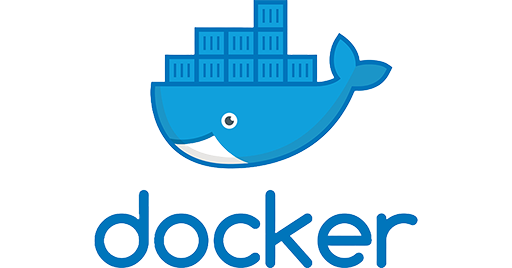
I have been using Docker Desktop for well over five years with very few problems besides the typical host-to-container mount performance issues. After a recent upgrade to Docker Desktop, I was surprised to notice that Docker Desktop would no longer start. It just reported a “Docker Desktop starting…” message and wouldn’t continue.
I tried the typically suggested approaches:
- Checked to ensure I was running the latest build of Docker Desktop
- Via the Docker > Troubleshoot menu
- Clean / Purge data
- Reset to factory defaults
- Uninstall
- Reinstall Docker
- Remove Docker from /Applications and empty Trash
- Restart the computer
- Reinstall Docker Desktop for Mac
Finally, after about two hours, a post on forums.docker.com by zcqian put me on the right track, so I wanted to promote it, along with some refinements and my complete solution (try parts or the complete procedure).
- Restart the computer, and make sure Docker is not running. If it is trying to start, then stop it and close it.
- Open your Applications folder and delete Docker, then empty the Trash (or
brew uninstall docker
). - Open Terminal and type:
rm -rf ~/Library/Group\ Containers/group.com.docker
rm -rf ~/Library/Containers/com.docker.*
rm -rf ~/Library/Application\ Support/Docker\ Desktop - Download Docker Desktop again, and copy
Docker
to your/Applications
folder (orbrew install --cask docker
). - Open Docker.
With any luck, you will now be back up and running again. Feel free to leave a comment if these steps change over time, and I will update this post accordingly.